This is a follow-up on the basic to-do list written by Sebastian Hette from theswiftguy youtube channel. You will need to follow the tutorial to make the app before you can start on this tutorial. It can be found here
How to do csv in ToDo App
1. Change your array to this
var list : NSMutableArray = ["Buy milk", "Run 5 miles", "Get Peter", "Plant my new plants"]
2. Create a new swift file called FileLoadModel . It should have this code in it
import UIKit
class FileLoadModel: NSObject {
var CSVfileloaded = false
var filePath = Bundle.main.path(forResource: "dataCSV", ofType: "csv")!
}
3. Under the Class FirstViewController declare a FileLoadModel variable. This variable calls the class we just made.
var myFileLoadModel = FileLoadModel()
4. Change the cell.textLabel?.text = list[indexPath.row] to
cell.textLabel?.text = String(describing: list[indexPath.row])
5. In the cell editing section, between the list.remove and the MyTableView.ReloadData add this: You will get an error but no problem
let fileName = "dataCSV"
let svc = SecondViewController()
svc.writeDataToFile(file: fileName, listArray: list)
6. After ViewDidAppear create a new function to find the path where the files will be saved by adding this code:
func getDirectoryPath() -> String {
let paths = NSSearchPathForDirectoriesInDomains(.documentDirectory, .userDomainMask, true)
let documentsDirectory = paths[0]
return documentsDirectory
}
7. Alright, now we need to add a whole heap of content in the viewDidLoad: *Once again you will get the SVC error, it is just because we have not declared it.
//These 3 lines allow the array to be loaded at the first screen by default.
myFileLoadModel.CSVfileloaded = true
let svc = SecondViewController()
svc.writeCSVtoList()
That is the FirstViewController sorted. We now have to fix up SecondViewController
8. We will declare our model at the top of the class as we did in the previous controller
var myFileLoadModel = FileLoadModel()
9. Create a new function to read the data from the file:
func readDataFromFile(file:String, type:String)-> String!{
let fileName = "/" + file + "." + type
let documentPath = getDirectoryPath() + fileName
do {
let contents = try String(contentsOfFile: documentPath)
return contents
} catch {
print("File Read Error for file \(fileName)")
return nil
}
}
10. Add
writeDataToFile(file: "dataCSV", listArray: list)
after you have appended the list. You will also need to add some code You will get an error as it is calling something we have not made yet
@IBAction func addItem(_ sender: AnyObject)
{
if (input.text != "")
{
list.add(input.text!)
writeDataToFile(file: "dataCSV", listArray: list)
input.text = ""
}
}
11. Create a new function to write data to file:
func writeDataToFile(file:String, listArray: NSMutableArray){
var csvText = ""
for i in 0..<listArray.count {
let newLine = "\(listArray[i])\n"
csvText.append(newLine)
}
let documentPath = getDirectoryPath() + "/dataCSV.csv"
do{
try csvText.write(toFile: documentPath, atomically: true, encoding: String.Encoding.utf8 )
} catch{
print("Failed to create file CSV")
}
myFileLoadModel.filePath = readDataFromFile(file: "dataCSV", type: "csv")
}
12. Now we need to parse the CSV to the list array
func writeCSVtoList() {
list = []
myFileLoadModel.filePath = readDataFromFile(file: "dataCSV", type: "csv")
let readings = (myFileLoadModel.filePath.components(separatedBy: "\n")) as [String]
for i in 0..<readings.count-1 {
list.add(readings[i])
}
}
Parsing the csv to the array
//parsing CSV
func writeCSVtoList() {
list = []
myFileLoadModel.filePath = readDataFromFile(file: "dataCSV", type: "csv")
let readings = (myFileLoadModel.filePath.components(separatedBy: "\n")) as [String]
for i in 0..<readings.count-1 {
list.add(readings[i])
}
}
13. Getting the directory path again
func getDirectoryPath() -> String {
let paths = NSSearchPathForDirectoriesInDomains(.documentDirectory, .userDomainMask, true)
let documentsDirectory = paths[0]
return documentsDirectory
}
You should now have the project parsing in .csv files into the array.
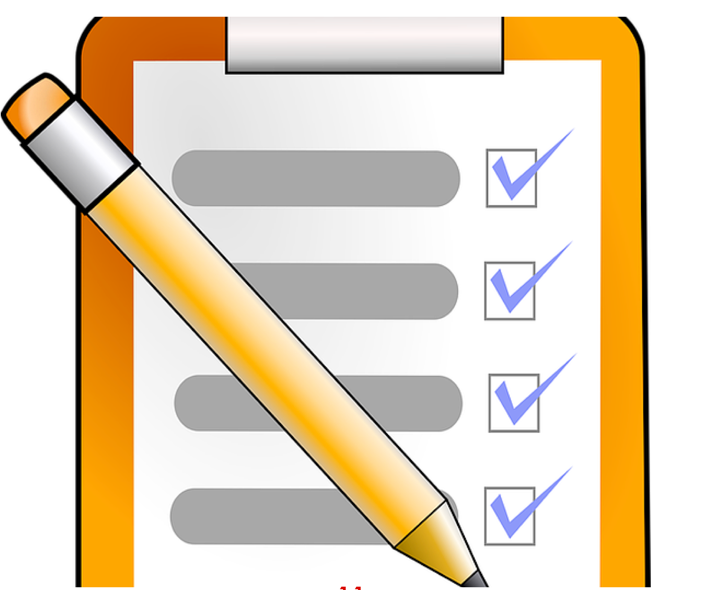
Comments